8Observer8 said:
You should to choose what game engine you are going to use in the future. For example, If you want to use Unreal Engine for 3D games and SFML for 3D games you should to study C++.
You can start to study pure C++ in console by creating simple games using SFML. It is very fun to study C++ with games. Install MinGW and lightweight editor VSCode: https://code.visualstudio.com/docs/languages/cpp
I will publish here my example in SFML and Makefile. Maybe it will be useful for someone.
Type the command: mingw32-make
I agree! SFML is a great tool to start building simple games and really understand what you are doing. Although if you are on windows I suggest using visual studio with SFML https://www.sfml-dev.org/tutorials/2.5/start-vc.php their documentation is great and you will be having a blast in no time!
On the other hand Unity and Unreal are great platforms to make developing a game a little less abstract and more WYSIWYG.
Bottom line is this:
it doesn't really matter in which language you program. You can do almost everything you can do in C++ in C# or Java. It all boils down which syntax you prefer. I came from PHP 4.x when I started so C++ was syntaxy (is that a word?) the closest language to dive into. But if I must i could just as easily write a function in Java or C#. As long as I am willing to take the time and learn the syntaxes and native functions of said language. The important part: the logic, ability to make an abstraction of the problem is all basically the same. What I am trying to say is this. If you can program in one language you can easily learn another language in the future.
One more piece of advice:
Don’t stay in ‘tutorial land’ too long. Once you know how to work with your platform of choice (for example C++ with SFML in visual studio) try to write a simple game like a ball moving from left to right. without watching a video or following a tutorial how to do the entire thing. Break the game into segments like so:
- Input
- Update
- Draw the current state
If it works great! Now try to make the ball move along a rectangle sprite. If that works add a control that makes the ball go up. If that works add gravity to make the ball fall on the rectangle sprite again. While doing this try to think of solutions to problems you encounter.
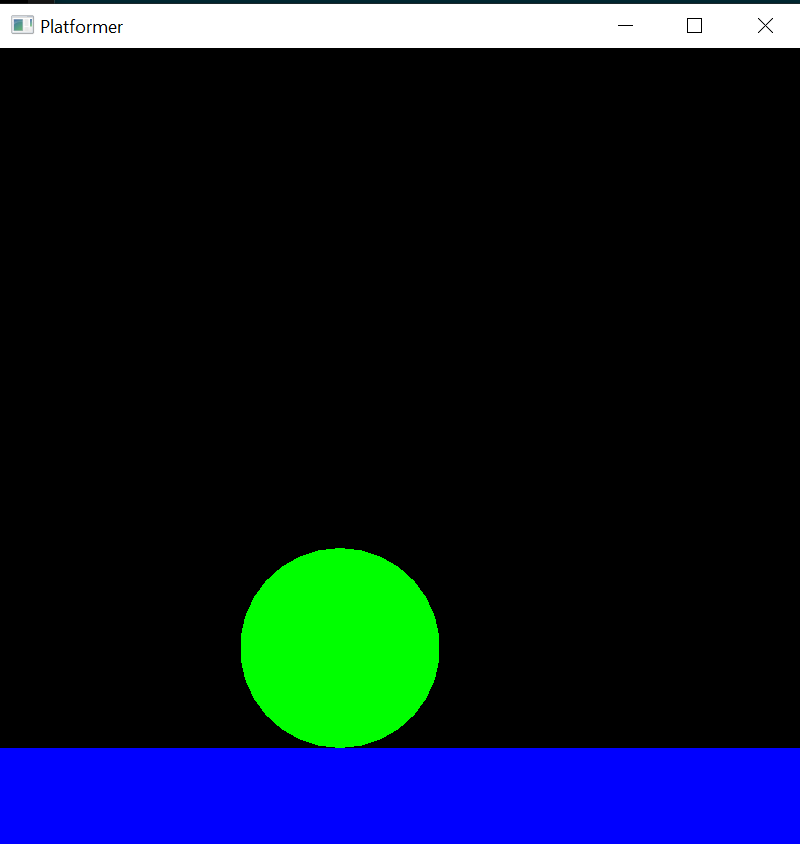
If you don’t know how to solve it? Look in the documentation, stack overflow or google other resources. Sooner or later you are going to get stuck on a problem. Don’t give up! But ask for help! Big chance somebody else has already encountered a similar problem and is willing to help you out. This way of learning to code games will be faster then just watching tutorials all day because you are more involved.
That said you have to learn the syntax somewhere I really love ‘the Cherno’ on YouTube for this:
https://www.youtube.com/watch?v=18c3MTX0PK0&list=PLlrATfBNZ98dudnM48yfGUldqGD0S4FFb
By the way the solution of the simple ball game:
#include <SFML/Graphics.hpp>
int mainWindowHeight = 800;
int mainWindowWidth = 800;
int main()
{
//set variables
sf::RenderWindow window(sf::VideoMode(mainWindowHeight, mainWindowWidth), "Platformer");
window.setFramerateLimit(60);
sf::CircleShape character(100.f);
character.setFillColor(sf::Color::Green);
character.setPosition(100,500);
sf::RectangleShape floorBit(sf::Vector2f(800,100));
floorBit.setFillColor(sf::Color::Blue);
floorBit.setPosition(0,700);
int bounce = 0;
int xVector = 0;
int jumpingAvailable = 1;
int duck = 0;
int yVector;
while(window.isOpen())
{
//input
xVector = 0;
if(duck == 1)
{
character.setScale(1,1);
duck = 0;
}
if(sf::Keyboard::isKeyPressed(sf::Keyboard::Space) && jumpingAvailable == 1)
{
bounce = -30;
jumpingAvailable = 0;
}
if(sf::Keyboard::isKeyPressed(sf::Keyboard::Left))
{
xVector = -10;
}
if(sf::Keyboard::isKeyPressed(sf::Keyboard::Right))
{
xVector = 10;
}
if(sf::Keyboard::isKeyPressed(sf::Keyboard::Down)&& duck == 0)
{
character.setScale(1,0.5f);
duck = 1;
}
sf::Event event;
while (window.pollEvent(event))
{
if(event.type == sf::Event::Closed)
{
window.close();
}
}
//update
yVector = 10 + bounce;
if(duck == 0)
{
if(character.getPosition().y +201 > floorBit.getPosition().y)
{
character.setPosition(character.getPosition().x, floorBit.getPosition().y-200);
yVector = bounce;
jumpingAvailable = 1;
}
}
else
{
if(character.getPosition().y +101 > floorBit.getPosition().y)
{
character.setPosition(character.getPosition().x, floorBit.getPosition().y-100);
yVector = bounce;
jumpingAvailable = 1;
}
}
character.move(xVector, yVector);
if(bounce < 0)
{
bounce += 1;
}
//check if character is in 'bounds'
if(character.getPosition().x < 0)
{
character.setPosition(0, character.getPosition().y);
}
if(character.getPosition().x >600 )
{
character.setPosition(600, character.getPosition().y);
}
//draw stuff each frame
window.clear();
window.draw(floorBit);
window.draw(character);
window.display();
}
return 0;
}